Reverse String
This Wednesday we will be looking at Reverse String. Another common interview question that shouldn’t be too challenging. As always, I suggest you give it a try or following along at: https://leetcode.com
The question today goes like this:
Write a function that reverses a string. The input string is given as an array of characters char[].
Do not allocate extra space for another array, you must do this by modifying the input array in-place with O(1) extra memory.
You may assume all the characters consist of printable ascii characters.
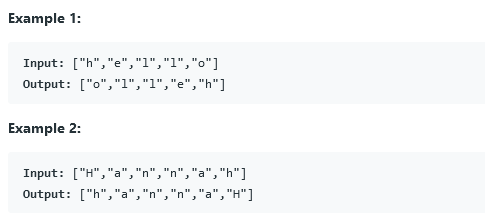
So the basic algorithm for Reverse String will look like this. We want to have a variable at the first index of our character array, and one at our last index. We will swap the two values and then move each pointer towards each other. When the lower pointer is greater than the higher pointer, we will stop.
Examples: “Hello”, we will set a variable (i) to zero (“H”) and another (j) to 4 (“O”). We will then switch them to get “oellH”. We will increment i and decrement j, then switch them again to get “olleH”, then we will increment i and decrement j. Then I will no longer be less than j, so we can stop, and we have our reversed string!
Code
First we will set the vales of our variables to the start and end of the character array.
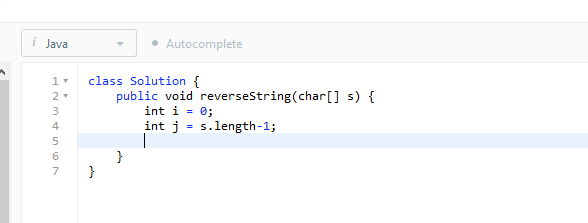
Then we will loop over the array and swap at each position, using a temp character.
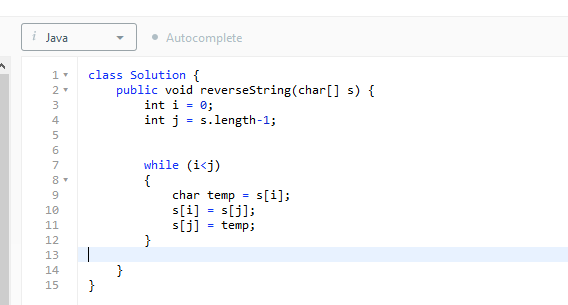
Don’t forget to increment and decrement our variables.
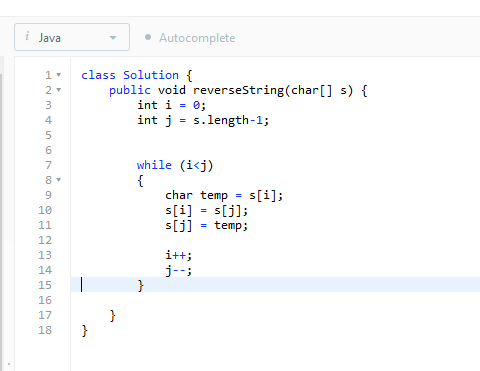
Tests
Then we are done! This method returns void so no need to return a value. We can submit it and see the results.
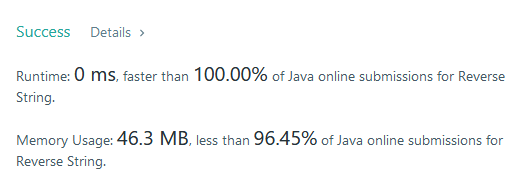
Great! In other examples of this question a String is passed in and expected to be returned. To see how we handle converting a character array to and from a string, check out this video showing the sample problem with a slightly different implementation.
For more tech blogs, subscribe to the code_marks news letter: http://eepurl.com/gZCMQz
1 Comment
Pingback: Coding interview question: Two Sums | Marked Code